7. NC Syntax and Semantics
An NC model consists of a header and a body, as seen below:
model( "model name" )
{
// body goes here
}
The header of a model consists of the word model, followed by one argument that is enclosed between parentheses. The argument is an arbitrary name in the form of a string that is between double quotes.
The body of the model follows immediately after the header and it is enclosed between opening and closing braces ("curly brackets").
The name of the model is purely for documentation purposes. It serves to help identify the model and it is passed on to the NEC-2 Card deck which you can export to other antenna programs.
As seen in the above example, you can insert a comment any place in the file (even outside the body). NC ignores the // (two slashes with no space in between) token and everything else until the end of a line.
Spaces, tabs, carriage returns and linefeeds are ignored by NC. You can place them where you think they would to improve legibility.
Types and Numbers
NC works with three data types. An integer number is called an int. A real or floating point number is known as a real, and a geometry element (e.g., a straight wire) is known as an element.
NC recognizes various forms of int and real constants.
int constants are integers or whole numbers, e.g. 1, -2, 13, 55, -377, etc.
A real constant contains a decimal point and it can take on fractional values, e.g., 3.1415926. Very large and very small numbers can be represented in the scientific notation. E.g., 1.2E-3 represents 1.2 times 10 to the power of minus three, or 0.0012.
Numbers that have dimensions (e.g., x, y, and z coordinate values of a wire element) are assumed to be in meters.
NC has some number conversion shortcuts to convert between units. If you place a singe double quote symbol right after a number (with no space between the number and the double quote) NC will assume that you want a value in units of inches. I.e., the constant 1.5" and the constant 0.0381 both express the dimension of 1.5 inches (0.0381 meters).
If you place a single quote right after a number, the number will be treated as one in units of feet by NC.
A u, n and p can be used to scale a numerical constant by 1E-6, 1E-9 and 1E-12. For example 10.5p is the same as writing 10.5*1E-12.
In addition, if you place a # (octathorpe or pound sign) directly before an integer constant, NC will interpret the dimension as the radius of a wire in the American Wire Gauge (AWG) tables.
Variables and Declarations
NC recognizes int, real and element variables. The use of variables can be used to illustrate the model more clearly, or they can be used to hold some intermediate value (e.g., the midpoint of a wire) or they can be used to hold values that you use in multiple places. It is easier and less error prone to assign a value to a variable and use that variable in different places. It is also easier to change the value of a variable (e.g., the height of the antenna) than to change it at multiple places while you are adjusting your antenna model.
Variables need to be "declared" before you use them. Variables can either be declared outside a model (global variables), or inside a model (local variables). We will later see why it is useful to maintain both global and local variables. Local variables are declared at the top of a function body. The following example shows a global variable called y and some local variables.
real y ;
model( "model name" )
{
real x, z ;
int segments ;
element driven ;
}
NC uses semicolons to separate statements and declarations. In the above, the semicolon after the variable z terminates the real declaration. The semicolon can then be followed by other declarations or statements. Commas are used to separate multiple variable declarations, as seen above between the variable x and the variable z.
Expressions
Constants and variables can be joined together to form expressions.
The simplest expression is a constant or variable by itself. E.g.,
1
2.71828
x
y
You can negate an expression with a - (minus) sign. You can pre-increment a scalar variable by using the ++ operator (e.g., ++n) or pre-decrement a variable using the -- operator, e.g., --n.
++n is the same as n= n+1.
You can multiply, divide and take the modulus (mod or remainder function) of two expressions using the *, / and % binary operators. In the case of the mod function, the result is the mod function of the integer parts of both operands.
For the multiply, divide and mod function, the expression is evaluated from left to right.
The next set of expressions is made of add and subtract operators. The operands are also evaluated from left to right, 1-2+3 evaluates to 2 instead of -4. However, the multiplicative operators (*,/ and %) have precedence and will override the left to right rule of the additive operator. I.e., for the expression a-b*c, the b*c term will be evaluated first and the result is subtracted from a.
The next set of expressions is the relational expressions with four operators < (less than), > (greater than), <= (less or equal) and >= (greater or equal). The result of a comparison is an integer value that is either 0 (for false) or 1 (for true). Between the relational operators, the evaluation is again from left to right, but the additive and multiplicative operators will take precedence (i.e, numbers will be added and multiplied before they are compared).
The next set of expressions is created from two equality operators == (for equal) and != (for not equal). The left to right rule is maintained but the previous operators have precedence.
The next set of operator are the logical and (&) and logical or (|) operator. Please note that unlike the C language, the are single characters, not && and ||.
Finally, lowest in precedence comes the assignment operator =. Thus, with an expression like a = b+c*d, the right hand side of the equal sign is evaluated first into a result, and the result then replaces the previous value of a.
You can also place expressions within parentheses to promote the precedence to a higher one. Since * (multiply) has a greater precedence than + (add), 1+2*4 will evaluate to 9 (the 2*4 will be evaluated first). However, (1+2)*4 will promote the precedence of the plus operator and the expression will be be evaluated as 12.
NC has a couple of built in constant variables, one is pi (3.14159...) and the other is c (299.7924...). The latter can be used to convert between frequency in MHz and wavelength in vacuum in meters.
You can use functions any place you can use variables. Functions have previously described in a previous chapter.
Statements
You can make an NC expression into a statement by appending a semicolon after it. The statements that are created this way can then be added into an model block. For example,
real y ;
model( "model name" )
{
real x, z, stretch ;
int segments ;
element driven ;
segments = 21 ;
x = 0 ;
y = 5.18 ;
z = 12 ;
stretch = 1.02 ;
driven = wire( x, y*stretch, z, x, -y*stretch, z, #14, segments ) ;
}
if Statements
You can conditionally execute a statement in NC by using an if statement. E.g.
if ( p ) x = 0 ;
In the above example, p is an int expression. The statement "x = 0 ;" is executed if p is non zero. Since the "less than" operator returns an int, zero if false and 1 if true, you can for example write
if ( x < 0 ) x = 0 ;
More complex conditions can be written, such as
if ( x < 0 | sqrt( x*x + y*y ) < 0.01 ) x = 0 ;
You can also follow an if statement optionally with an else condition, e.g.,
if ( x < 0 ) x = 0 ; else x = 1 ;
Iterative Statements
You can repeat a statement for a number of times by using the repeat statement. E.g.,
repeat ( 5 ) x = x + 0.1 ;
In this simple example, x = x + 0.1 will be repeated 5 times. The value of x will increase by 0.5 at the end of the repeat statement.
The repeat clause (5 in the above example) can be any integer expression, e.g.,
repeat ( n+5 ) x = x + 0.1 ;
Another iterative statement that you can use in NC is the while statement. E.g.,
x = 0.0 ;
while ( x < 1.0 ) x = x + 0.15 ;
In this simple example, when the while statement is done, x will take on the value of 1.05.
Compound Statements
You can group an ordered list of statements within braces (curly bracket) to form a compound statement. The rest of NC will treat this as a single statement. This is especially useful for selective and iterative statements, e.g.,
if ( x == 0 ) {
y = 0 ;
z = 1 ;
}
break Statement
Please note that the while statement uses a logical expression as the loop condition. As long as the expression in the while clause is non-zero, the while loop continues executing.
The repeat statement, on the other hand, takes an integer count as its clause. This count is computed and fixed at the beginning of the repeat loop, and the loop runs for that predetermined number of times. If the count evaluates to 0 or a negative integer, the repeat statement will not run.
You can also break out of a while or repeat loop before the above conditions are met. To do that, you use a break statement. The following example will behave precisely the same as the while example above.
x = 0.0 ;
while ( 1 ) {
x = x + 0.15 ;
if ( x >= 1.0 ) break ;
}
The "while ( 1 )" will cause the loop to execute indefinitely. However, the conditional statement will cause you to break out of the loop when x is greater or equal to 1.0.
Forced Break
When you press Run, you will see a second button called Stop appear in the NC window:
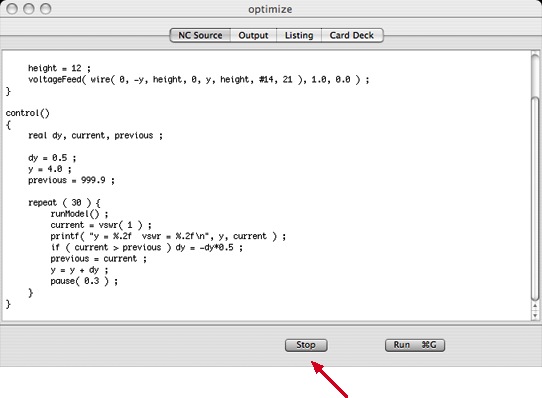
Clicking on Stop will break out of all repeat and while loops.
The Stop button will be hidden again once the Run has completed.
Next: Writing Optimizing Loops